Data consistency and performance in web applciation
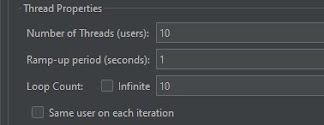
Introduction In this article we are going to look at various implementations of bank transfer in a web applciation API taking into consideration data consistency and performance. Technologies and software I am using in examples below are: PostgreSQL, Java 17, JDBC, Spark Java, JMeter, Postman, IntelliJ Approach 1 - plain SQLs We start by creating a following piece of code to perform a bank transfer: In terms of DB operations we can write it in following way: SELECT source account balance SELECT target account balance UPDATE source account balance UPDATE target account balance We can test how will it behave when there are multiple users performing transfers concurrently. In my case I am going to place this code in a simple Jetty server and use JMeter to simulate the traffic. Here is the JMeter load testing thread config (please note that same user on each iteration is unchecked): with web server request config: and payload: As you can see we will perform 100 POST request